Journey into OpenGL: the Framebuffer and Depth Buffer
JiGL
In the previous page, I mentioned the "screen" as a huge matrix of pixels. Formally, it is more complicated.
OpenGL defines the Framebuffer, which is composed of multiple buffers the size of the screen. the buffer, which holds the pixels shown on your monitor, is called the front buffer. the buffer which you draw to is the back buffer. the technique of using two buffers is called double buffering. While doing away with the back buffer is possible, it causes routine clashing between the graphics card and the software, as the card may read the single buffer before the software is done drawing. This causes a very annoying flickering, which harms the user experience. GLFW by default grants you double buffering. That is the meaning of the glfwSwapBuffers
call in the main rendering loop: to swap the front and back buffers, finally presenting what you have had drawn.
Those are the color buffers. Besides those, there are other powerful buffers such as the depth buffer or the stencil buffer.
Let us go over this example, which produces unintuitive results. I will have it draw two overlapping triangles with custom depth, the second of which is deeper. They will also have different colors.
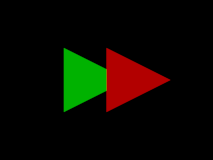
glBegin(GL_TRIANGLES);
glColor3f(0, 0.7, 0);
glVertex3f(-0.4, +0.4, 0);
glVertex3f(-0.4, -0.4, 0);
glVertex3f(+0.2, 0, 0);
glColor3f(0.7, 0, 0);
glVertex3f(0, +0.4, 0.5);
glVertex3f(0, -0.4, 0.5);
glVertex3f(+0.6, 0, 0.5);
glEnd();
Notice that the second, red triangle appears to be in front of the first, despite being deeper. This is because OpenGL does not remember each triangle you send it. the ray analogy I had used in the previous article is applicable only to projections, as OpenGL does not actually trace rays (not on its own, anyway). Whatever you send it draws immediately, as if painting. This may seem strange, but doing it otherwise would demand too much from the graphics accelerator. But then, what is the purpose of depth?
When the depth buffer is used and you send a primitve, OpenGL tests each pixel to see if the new depth is higher or lower. This way it chooses a fragment to throw away or keep. If kept, the depth buffer and color buffer are written into. This depth buffer is by default disabled, so let us enable it: before your glBegin, have glEnable(GL_DEPTH_TEST);
. We shall also need to clear the depth buffer each frame, in addition to the color buffer. After this, you should have the expected result.
glClear(GL_COLOR_DEPTH_BIT);
glClear(GL_DEPTH_BUFFER_BIT);
glEnable(GL_DEPTH_TEST);
glBegin(GL_TRIANGLES);
glColor3f(0, 0.7, 0);
glVertex3f(-0.4, +0.4, 0);
glVertex3f(-0.4, -0.4, 0);
glVertex3f(+0.2, 0, 0);
glColor3f(0.7, 0, 0);
glVertex3f(0, +0.4, 0.5);
glVertex3f(0, -0.4, 0.5);
glVertex3f(+0.6, 0, 0.5);
glEnd();
Remember I said how glClear
accepts multiple arguments. To minimize our calls to OpenGL, we can and should take advantage of that: glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
.
This depth buffer (also called the Z-buffer) is what allows us to reduce load on the accelerator and, thanks to its parallel nature, draw 3D scenes quickly. Without it, the program would have to manually sort shapes by depth which would take a lot of time, as was the case on the PS1. Crash Bandicoot, for instance, avoided the problem by having the camera point in one direction only. This allowed it to sort shapes only once. Well done to them but that isn't always possible.
In 2D the situation is different. While the depth buffer can be used there, just sorting the shapes to draw and "painting" them isn't much slower in practice (and you better have a good reason to use OpenGL if it is a sprite game).
From now on we shall assume depth testing to always be on.
Here are more flashcards. I remind you again to write down answers on paper before clicking. It's easy to only think you've got it.
How are 3D scenes drawn with proper depth?
By employing a depth buffer, we can tell the depth the scene at any point on the screen. Using that information we decide whether to not draw another shape, depending on which is closer.
How is a scene rendered without a depth buffer?
Like in painting, the last shape drawn is what is seen.
What is a Framebuffer?
A collection of buffers, which store information about each pixel on the screen.
What is a color buffer?
A buffer that stores color information.
What is double buffering?
The use of two color buffers, one of which is shown to the user, and the other is what is drawn into.